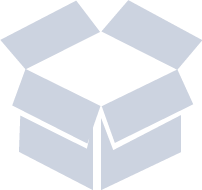
DES_CRYPT(3) DES_CRYPT(3) NAME des_read_password, des_read_2password, des_string_to_key, des_string_to_2key, des_read_pw_string, des_random_key, des_set_key, des_key_sched, des_ecb_encrypt, des_3ecb_encrypt, des_cbc_encrypt, des_3cbc_encrypt, des_pcbc_encrypt, des_cfb_encrypt, des_ofb_encrypt, des_cbc_cksum, des_quad_cksum, des_enc_read, des_enc_write, des_set_odd_parity, des_is_weak_key, crypt - (non USA) DES encryption SYNOPSIS #include <des.h> int des_read_password(key,prompt,verify) des_cblock *key; char *prompt; int verify; int des_read_2password(key1,key2,prompt,verify) des_cblock *key1,*key2; char *prompt; int verify; int des_string_to_key(str,key) char *str; des_cblock *key; int des_string_to_2keys(str,key1,key2) char *str; des_cblock *key1,*key2; int des_read_pw_string(buf,length,prompt,verify) char *buf; int length; char *prompt; int verify; int des_random_key(key) des_cblock *key; int des_set_key(key,schedule) des_cblock *key; des_key_schedule schedule; int des_key_sched(key,schedule) des_cblock *key; des_key_schedule schedule; int des_ecb_encrypt(input,output,schedule,encrypt) des_cblock *input; des_cblock *output; des_key_schedule schedule; int encrypt; - 1 - Formatted: April 26, 2024 DES_CRYPT(3) DES_CRYPT(3) int des_3ecb_encrypt(input,output,ks1,ks2,encrypt) des_cblock *input; des_cblock *output; des_key_schedule ks1,ks2; int encrypt; int des_cbc_encrypt(input,output,length,schedule,ivec,encrypt) des_cblock *input; des_cblock *output; long length; des_key_schedule schedule; des_cblock *ivec; int encrypt; int des_3cbc_encrypt(input,output,length,sk1,sk2,ivec1,ivec2,encrypt) des_cblock *input; des_cblock *output; long length; des_key_schedule sk1; des_key_schedule sk2; des_cblock *ivec1; des_cblock *ivec2; int encrypt; int des_pcbc_encrypt(input,output,length,schedule,ivec,encrypt) des_cblock *input; des_cblock *output; long length; des_key_schedule schedule; des_cblock *ivec; int encrypt; int des_cfb_encrypt(input,output,numbits,length,schedule,ivec,encrypt) unsigned char *input; unsigned char *output; int numbits; long length; des_key_schedule schedule; des_cblock *ivec; int encrypt; int des_ofb_encrypt(input,output,numbits,length,schedule,ivec) unsigned char *input,*output; int numbits; long length; des_key_schedule schedule; des_cblock *ivec; unsigned long des_cbc_cksum(input,output,length,schedule,ivec) des_cblock *input; des_cblock *output; - 2 - Formatted: April 26, 2024 DES_CRYPT(3) DES_CRYPT(3) long length; des_key_schedule schedule; des_cblock *ivec; unsigned long des_quad_cksum(input,output,length,out_count,seed) des_cblock *input; des_cblock *output; long length; int out_count; des_cblock *seed; int des_check_key; int des_enc_read(fd,buf,len,sched,iv) int fd; char *buf; int len; des_key_schedule sched; des_cblock *iv; int des_enc_write(fd,buf,len,sched,iv) int fd; char *buf; int len; des_key_schedule sched; des_cblock *iv; extern int des_rw_mode; void des_set_odd_parity(key) des_cblock *key; int des_is_weak_key(key) des_cblock *key; char *crypt(passwd,salt) char *passwd; char *salt; DESCRIPTION This library contains a fast implementation of the DES encryption algorithm. There are two phases to the use of DES encryption. The first is the generation of a des_key_schedule from a key, the second is the actual encryption. A des key is of type des_cblock. This type is made from 8 characters with odd parity. The least significant bit in the character is the parity bit. The key schedule is an expanded form of the key; it is used to speed the encryption process. - 3 - Formatted: April 26, 2024 DES_CRYPT(3) DES_CRYPT(3) des_read_password writes the string specified by prompt to the standard output, turns off echo and reads an input string from standard input until terminated with a newline. If verify is non- zero, it prompts and reads the input again and verifies that both entered passwords are the same. The entered string is converted into a des key by using the des_string_to_key routine. The new key is placed in the des_cblock that was passed (by reference) to the routine. If there were no errors, des_read_password returns 0, -1 is returned if there was a terminal error and 1 is returned for any other error. des_read_2password operates in the same way as des_read_password except that it generates 2 keys by using the des_string_to_2key function. des_read_pw_string is called by des_read_password to read and verify a string from a terminal device. The string is returned in buf. The size of buf is passed to the routine via the length parameter. des_string_to_key converts a string into a valid des key. des_string_to_2key converts a string into 2 valid des keys. This routine is best suited for used to generate keys for use with des_3ecb_encrypt. des_random_key returns a random key that is made of a combination of process id, time and an increasing counter. Before a des key can be used it is converted into a des_key_schedule via the des_set_key routine. If the des_check_key flag is non-zero, des_set_key will check that the key passed is of odd parity and is not a week or semi-weak key. If the parity is wrong, then -1 is returned. If the key is a weak key, then -2 is returned. If an error is returned, the key schedule is not generated. des_key_sched is another name for the des_set_key function. The following routines mostly operate on an input and output stream of des_cblock's. des_ecb_encrypt is the basic DES encryption routine that encrypts or decrypts a single 8-byte des_cblock in electronic code book mode. It always transforms the input data, pointed to by input, into the output data, pointed to by the output argument. If the encrypt argument is non-zero (DES_ENCRYPT), the input (cleartext) is encrypted in to the output (ciphertext) using the key_schedule specified by the schedule argument, previously set via des_set_key. If encrypt is zero (DES_DECRYPT), the input (now ciphertext) is decrypted into the output (now cleartext). Input and output may overlap. No meaningful value is returned. - 4 - Formatted: April 26, 2024 DES_CRYPT(3) DES_CRYPT(3) des_3ecb_encrypt encrypts/decrypts the input block by using triple ecb DES encryption. This involves encrypting the input with ks1, decryption with the key schedule ks2, and then encryption with the first again. This routine greatly reduces the chances of brute force breaking of DES and has the advantage of if ks1 and ks2 are the same, it is equivalent to just encryption using ecb mode and ks1 as the key. des_cbc_encrypt encrypts/decrypts using the cipher-block-chaining mode of DES. If the encrypt argument is non-zero, the routine cipher- block-chain encrypts the cleartext data pointed to by the input argument into the ciphertext pointed to by the output argument, using the key schedule provided by the schedule argument, and initialisation vector provided by the ivec argument. If the length argument is not an integral multiple of eight bytes, the last block is copied to a temporary area and zero filled. The output is always an integral multiple of eight bytes. To make multiple cbc encrypt calls on a large amount of data appear to be one des_cbc_encrypt call, the ivec of subsequent calls should be the last 8 bytes of the output. des_3cbc_encrypt encrypts/decrypts the input block by using triple cbc DES encryption. This involves encrypting the input with key schedule ks1, decryption with the key schedule ks2, and then encryption with the first again. 2 initialisation vectors are required, ivec1 and ivec2. Unlike des_cbc_encrypt, these initialisation vectors are modified by the subroutine. This routine greatly reduces the chances of brute force breaking of DES and has the advantage of if ks1 and ks2 are the same, it is equivalent to just encryption using cbc mode and ks1 as the key. des_pcbc_encrypt encrypt/decrypts using a modified block chaining mode. It provides better error propagation characteristics than cbc encryption. des_cfb_encrypt encrypt/decrypts using cipher feedback mode. This method takes an array of characters as input and outputs and array of characters. It does not require any padding to 8 character groups. Note: the ivec variable is changed and the new changed value needs to be passed to the next call to this function. Since this function runs a complete DES ecb encryption per numbits, this function is only suggested for use when sending small numbers of characters. des_ofb_encrypt encrypt using output feedback mode. This method takes an array of characters as input and outputs and array of characters. It does not require any padding to 8 character groups. Note: the ivec variable is changed and the new changed value needs to be passed to the next call to this function. Since this function runs a complete DES ecb encryption per numbits, this function is only suggested for use when sending small numbers of characters. des_cbc_cksum produces an 8 byte checksum based on the input stream (via cbc encryption). The last 4 bytes of the checksum is returned - 5 - Formatted: April 26, 2024 DES_CRYPT(3) DES_CRYPT(3) and the complete 8 bytes is placed in output. des_quad_cksum returns a 4 byte checksum from the input bytes. The algorithm can be iterated over the input, depending on out_count, 1, 2, 3 or 4 times. If output is non-NULL, the 8 bytes generated by each pass are written into output. des_enc_write is used to write len bytes to file descriptor fd from buffer buf. The data is encrypted via pcbc_encrypt (default) using sched for the key and iv as a starting vector. The actual data send down fd consists of 4 bytes (in network byte order) containing the length of the following encrypted data. The encrypted data then follows, padded with random data out to a multiple of 8 bytes. des_enc_read is used to read len bytes from file descriptor fd into buffer buf. The data being read from fd is assumed to have come from des_enc_write and is decrypted using sched for the key schedule and iv for the initial vector. The des_enc_read/des_enc_write pair can be used to read/write to files, pipes and sockets. I have used them in implementing a version of rlogin in which all data is encrypted. des_rw_mode is used to specify the encryption mode to use with des_enc_read and des_end_write. If set to DES_PCBC_MODE (the default), des_pcbc_encrypt is used. If set to DES_CBC_MODE des_cbc_encrypt is used. These two routines and the variable are not part of the normal MIT library. des_set_odd_parity sets the parity of the passed key to odd. This routine is not part of the standard MIT library. des_is_weak_key returns 1 is the passed key is a weak key (pick again :-), 0 if it is ok. This routine is not part of the standard MIT library. crypt is a replacement for the normal system crypt. It is much faster than the system crypt. FILES /usr/include/des.h /usr/lib/libdes.a The encryption routines have been tested on 16bit, 32bit and 64bit machines of various endian and even works under VMS. BUGS If you think this manual is sparse, read the des_crypt(3) manual from the MIT kerberos (or bones outside of the USA) distribution. des_cfb_encrypt and des_ofb_encrypt operates on input of 8 bits. What this means is that if you set numbits to 12, and length to 2, the first 12 bits will come from the 1st input byte and the low half of - 6 - Formatted: April 26, 2024 DES_CRYPT(3) DES_CRYPT(3) the second input byte. The second 12 bits will have the low 8 bits taken from the 3rd input byte and the top 4 bits taken from the 4th input byte. The same holds for output. This function has been implemented this way because most people will be using a multiple of 8 and because once you get into pulling bytes input bytes apart things get ugly! des_read_pw_string is the most machine/OS dependent function and normally generates the most problems when porting this code. des_string_to_key is probably different from the MIT version since there are lots of fun ways to implement one-way encryption of a text string. The routines are optimised for 32 bit machines and so are not efficient on IBM PCs. NOTE: extensive work has been done on this library since this document was origionally written. Please try to read des.doc from the libdes distribution since it is far more upto date and documents more of the functions. Libdes is now also being shipped as part of SSLeay, a general cryptographic library that amonst other things implements netscapes SSL protocoll. The most recent version can be found in SSLeay distributions. AUTHOR Eric Young (eay@mincom.oz.au or eay@psych.psy.uq.oz.au) - 7 - Formatted: April 26, 2024