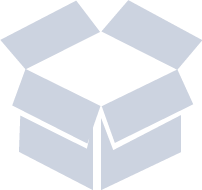
provides a simplified, portable interface to several low-level networking routines, including network address manipulation, kernel cache and table lookup and manipulation, network firewalling, network interface lookup and manipulation, and raw IP packet and Ethernet frame transmission. It is intended to complement the functionality provided by In addition, also provides platform-independent definitions of various network protocol formats and values for portable low-level network programming, as well as a simple binary buffer handling API. Network addresses are described by the following structure: struct addr { uint16_t addr_type; uint16_t addr_bits; union { eth_addr_t __eth; ip_addr_t __ip; ip6_addr_t __ip6; uint8_t __data8[16]; uint16_t __data16[8]; uint32_t __data32[4]; } __addr_u; }; #define addr_eth __addr_u.__eth #define addr_ip __addr_u.__ip #define addr_ip6 __addr_u.__ip6 #define addr_data8 __addr_u.__data8 #define addr_data16 __addr_u.__data16 #define addr_data32 __addr_u.__data32 The following values are defined for #define ADDR_TYPE_NONE 0 /* No address set */ #define ADDR_TYPE_ETH 1 /* Ethernet */ #define ADDR_TYPE_IP 2 /* Internet Protocol v4 */ #define ADDR_TYPE_IP6 3 /* Internet Protocol v6 */ The field denotes the length of the network mask in bits. compares network addresses and returning an integer less than, equal to, or greater than zero if is found, respectively, to be less than, equal to, or greater than Both addresses must be of the same address type. computes the broadcast address for the network specified in and writes it into computes the network address for the network specified in and writes it into converts an address from network format to a string. converts an address (or hostname) from a string to network format. converts an address from network format to a string, returning a pointer to the result in static memory. is a synonym for converts an address from network format to the appropriate struct sockaddr. converts an address from a struct sockaddr to network format. converts a network mask length to a network mask specified as a struct sockaddr. converts a network mask specified in a struct sockaddr to a network mask length. converts a network mask length to a network mask in network byte order. converts a network mask in network byte order to a network mask length. ARP cache entries are described by the following structure: struct arp_entry { struct addr arp_pa; /* protocol address */ struct addr arp_ha; /* hardware address */ }; is used to obtain a handle to access the kernel cache. adds a new ARP deletes the ARP for the protocol address specified by retrieves the ARP for the protocol address specified by iterates over the kernel cache, invoking the specified with each and the context passed to closes the specified handle. Binary buffers are described by the following structure: typedef struct blob { u_char *base; /* start of data */ int off; /* offset into data */ int end; /* end of data */ int size; /* size of allocation */ } blob_t; is used to allocate a new dynamic binary buffer, returning NULL on failure. reads bytes from the current offset in blob into returning the total number of bytes read, or -1 on failure. writes bytes from to blob advancing the current offset. It returns the number of bytes written, or -1 on failure. repositions the offset within blob to according to the directive (see for details), returning the new absolute offset, or -1 on failure. returns the offset of the first occurence in blob of the specified of length or -1 on failure. returns the offset of the last occurence in blob of the specified of length or -1 on failure. converts and writes, and reads and converts data in blob according to the given format as described below, returning 0 on success, and -1 on failure. The format string is composed of zero or more directives: ordinary characters (not ), which are copied to / read from the blob, and conversion specifications, each of which results in reading / writing zero or more subsequent arguments. Each conversion specification is introduced by the character and may be prefixed by length specifier. The arguments must correspond properly (after type promotion) with the length and conversion specifiers. The length specifier is either a a decimal digit string specifying the length of the following argument, or the literal character indicating that the length should be read from an integer argument for the argument following it. The conversion specifiers and their meanings are: An unsigned 32-bit integer in network byte order. An unsigned 16-bit integer in network byte order. A binary buffer (length specifier required). An unsigned character. An unsigned 32-bit integer in host byte order. An unsigned 16-bit integer in host byte order. A C-style null-terminated string, whose maximum length must be specified when unpacking. Custom conversion routines and their specifiers may be registered via currently undocumented. prints bytes of the contents of blob from the current offset in the specified currently only is available. deallocates the memory associated with blob and returns NULL. is used to obtain a handle to transmit raw Ethernet frames via the specified network retrieves the hardware MAC address for the interface specified by configures the hardware MAC address for the interface specified by transmits bytes of the Ethernet frame pointed to by closes the specified handle. Firewall rules are described by the following structure: struct fw_rule { char fw_device[INTF_NAME_LEN]; /* interface name */ uint8_t fw_op; /* operation */ uint8_t fw_dir; /* direction */ uint8_t fw_proto; /* IP protocol */ struct addr fw_src; /* src address / net */ struct addr fw_dst; /* dst address / net */ uint16_t fw_sport[2]; /* range / ICMP type */ uint16_t fw_dport[2]; /* range / ICMP code */ }; The following values are defined for #define FW_OP_ALLOW 1 #define FW_OP_BLOCK 2 The following values are defined for #define FW_DIR_IN 1 #define FW_DIR_OUT 2 is used to obtain a handle to access the local network firewall configuration. adds the specified firewall deletes the specified firewall iterates over the active firewall ruleset, invoking the specified with each and the context passed to closes the specified handle. Network interface information is described by the following structure: #define INTF_NAME_LEN 16 struct intf_entry { u_int intf_len; /* length of entry */ char intf_name[INTF_NAME_LEN]; /* interface name */ u_short intf_type; /* interface type (r/o) */ u_short intf_flags; /* interface flags */ u_int intf_mtu; /* interface MTU */ struct addr intf_addr; /* interface address */ struct addr intf_dst_addr; /* point-to-point dst */ struct addr intf_link_addr; /* link-layer address */ u_int intf_alias_num; /* number of aliases */ struct addr intf_alias_addrs __flexarr; /* array of aliases */ }; The following bitmask values are defined for #define INTF_TYPE_OTHER 1 /* other */ #define INTF_TYPE_ETH 6 /* Ethernet */ #define INTF_TYPE_LOOPBACK 24 /* software loopback */ #define INTF_TYPE_TUN 53 /* proprietary virtual/internal */ The following bitmask values are defined for #define INTF_FLAG_UP 0x01 /* enable interface */ #define INTF_FLAG_LOOPBACK 0x02 /* is a loopback net (r/o) */ #define INTF_FLAG_POINTOPOINT 0x04 /* point-to-point link (r/o) */ #define INTF_FLAG_NOARP 0x08 /* disable ARP */ #define INTF_FLAG_BROADCAST 0x10 /* supports broadcast (r/o) */ #define INTF_FLAG_MULTICAST 0x20 /* supports multicast (r/o) */ is used to obtain a handle to access the network interface configuration. retrieves an interface configuration keyed on For all functions, should be set to the size of the buffer pointed to by (usually sizeof(struct intf_entry), but should be larger to accomodate any interface alias addresses. retrieves the configuration for the interface whose primary address matches the specified retrieves the configuration for the best interface with which to reach the specified sets the interface configuration iterates over all network interfaces, invoking the specified with each interface configuration and the context passed to closes the specified handle. is used to obtain a handle to transmit raw IP packets, routed by the kernel. adds the header option for the protocol specified by of length and appends it to the appropriate header of the IP packet contained in of size shifting any existing payload and adding NOPs to pad the option to a word boundary if necessary. sets the IP checksum and any appropriate transport protocol checksum for the IP packet pointed to by of length transmits bytes of the IP packet pointed to by closes the specified handle. sets the appropriate transport protocol checksum for the IPv6 packet pointed to by of length is used to obtain a handle for fast, cryptographically strong pseudo-random number generation. The starting seed is derived from the system random data source device (if one exists), or from the current time and random stack contents. re-initializes the PRNG to start from a known value, useful in generating repeatable sequences. writes random bytes into adds bytes of entropy data from into the random mix. and return 8, 16, and 32-bit unsigned random values, respectively. randomly shuffles an array of elements of bytes, starting at closes the specified handle. Routing table entries are described by the following structure: struct route_entry { struct addr route_dst; /* destination address */ struct addr route_gw; /* gateway address */ }; is used to obtain a handle to access the kernel table. adds a new routing table deletes the routing table for the destination prefix specified by retrieves the routing table for the destination prefix specified by iterates over the kernel table, invoking the specified with each and the context passed to closes the specified handle. is used to obtain a handle to a network tunnel interface, to which IP packets destined for are delivered (with source addresses rewritten to ), where they may be read by a userland process and processed as desired. IP packets written back to the handle are injected into the kernel networking subsystem. returns a file descriptor associated with the tunnel handle, suitable for returns a pointer to the tunnel interface name. submits a packet to the kernel networking subsystem for delivery. reads the next packet delivered to the tunnel interface. closes the specified handle. returns a pointer to the argument, or NULL on failure. returns a pointer to a static memory area containing the printable address, or NULL on failure. and return a valid handle on success, or NULL on failure. and always return NULL. and return the length of the datagram successfully sent, or -1 on failure. and return the status of their routines. Any non-zero return from a will cause the loop to exit immediately. returns the length of the inserted option (which may have been padded with NOPs for memory alignment) or -1 on failure. and return 8, 16, and 32-bit unsigned random values, respectively. All other routines return 0 on success, or -1 on failure. Dug Song